In the article for step 5, we began to get into the main functions of this read-only REST service. We now know how to gather unique counties and regions from our database, and will now retrieve the data based on region/county selections or partial name entered,
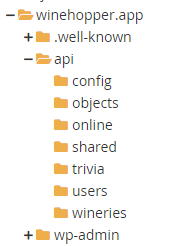
We are still working in the wineries folder and will show complete code for wineries_by_region.php and then review the slight code modifications made to wineries_by_county.php and wineries_by_name.php
<?php
// required headers
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: GET");
header("Access-Control-Allow-Credentials: true");
header('Content-Type: application/json');
// include database and object files
include_once '../config/database.php';
include_once '../objects/wineries.php';
$cred = 0;
$user = $_SERVER['PHP_AUTH_USER'];
$pwd = $_SERVER['PHP_AUTH_PW'];
if(sha1('* user *') == $user and sha1('* pwd *') == $pwd)
$cred = 1;
if($cred == 0) {
// set response code - 403 Not found
http_response_code(401);
// tell the user product does not exist
echo json_encode(array("message" => "unauthorized"));
die;
}
// get database connection
$database = new Database();
$db = $database->getConnection();
// prepare product object
$wineries = new Wineries($db);
// set ID property of record to read
$search = isset($_GET['region']) ? $_GET['region'] : die();
$state = isset($_GET['state']) ? $_GET['state'] : die();
// read the details of product to be edited
$stmt = $wineries->searchByRegion($search, $state);
$num = $stmt->rowCount();
if($num > 0){
// create array
$winery_arr = array();
$winery_arr["records"] = array();
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)){
extract($row);
$winery_item = array(
"id" => $id,
"name" => $Name,
"address" => $Address,
"city" => $City,
"state" => $State,
"zip" => $Zip,
"county" => $County,
"website" => $Website,
"phone" => $Phone,
"type" => $Type,
"tastings" => $Tastings,
"tours" => $Tours,
"region" => $Region,
"latitude" => $Latitude,
"longitude" => $Longitude,
"tripadvisor" => $Tripadvisor,
"googlemaps" => $Googlemaps,
"yelp" => $Yelp,
"twitter" => $Twitter,
"facebook" => $Facebook,
"instagram" => $Instagram,
"youtube" => $Youtube,
"weburl" => $Weburl
);
array_push($winery_arr["records"], $winery_item);
}
// set response code - 200 OK
http_response_code(200);
// make it json format
echo json_encode($winery_arr);
}
else{
// set response code - 404 Not found
http_response_code(404);
// tell the user product does not exist
echo json_encode(array("message" => "no wineries found"));
}
?>
Here we have the same basic approach seen in step 4, 5 and others of authorizing and connecting to the database. We started with this procedure since the distinction from the others is the use of two parameters – region and state. Combination of search fields will be necessary when the same name falls into two or more classifications. In this case, we will have the Central and Mountain regions in multiple states.
We call the searchByRegion function and feed it both of those parameters. The SQL command used by that function searches for the records matching state and region. We then send the fields shown in the returned array back to our calling web page using encoded JSON.
So what is the difference for wineries_by_county.php?
$search = isset($_GET['county']) ? $_GET['county'] : die();
$state = isset($_GET['state']) ? $_GET['state'] : die();
$stmt = $wineries->selectByCounty($search, $state);
Only slight differences in the check that we have the necessary parameters of county and state and the call to selectByCounty function using those parameters. All of the other PHP code is exactly the same.
In the current use case (records from NC/VA/NY), we don’t have any counties that exist in multiple states, although this parameter is included to account for that possibility at a later time.
How about wineries_by_name.php? Only slight differences again.
$search = isset($_GET['name']) ? $_GET['name'] : die();
$stmt = $wineries->searchByName($search);
Since we only need the partial name parameter, that is the only verification being done before continuing. We then execute the searchByName function to retrieve the same fields for names using the SQL LIKE clause (%name%).
One procedure not mentioned, and not used for this web page, is wineries_by_id.php. Unlike the other processes, this will use an ID parameter to return a single record equal to that ID.
$search = isset($_GET['id']) ? $_GET['id'] : die();
$stmt = $wineries->searchByID($search);
Following these steps (1-6), you have the basis for creating a PHP REST service which can be used to send records to your Android or iOS mobile app, database-enabled web page, and any other focus that you may dream up.
Next, we get to review designing the supporting database structure in MySQL using the PHPMyAdmin interface.