In the article for step 3, we looked at the shared directory and the getPaging function. Although these are not used in our final solution – Wet Ur Whis(tel), we have gained the experience of creating shared functions. Next we will cover the trivia folder and the PHP code used to load a trivia question, hint and answer using ID as the parameter.
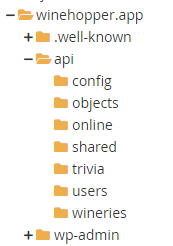
In the trivia folder we have one file called questions_by_id.php. The code which loads question data is shown below.
<?php
// required headers
header("Access-Control-Allow-Origin: *");
header("Access-Control-Allow-Headers: access");
header("Access-Control-Allow-Methods: GET");
header("Access-Control-Allow-Credentials: true");
header('Content-Type: application/json');
// include database and object files
include_once '../config/database.php';
include_once '../objects/trivia.php';
$cred = 0;
$user = $_SERVER['PHP_AUTH_USER'];
$pwd = $_SERVER['PHP_AUTH_PW'];
if(sha1('* encrypted user name goes here *') == $user and sha1('* encrypted password goes here *') == $pwd)
$cred = 1;
if($cred == 0) {
// set response code - 403 Not found
http_response_code(401);
// tell the user product does not exist
echo json_encode(array("message" => "unauthorized"));
die;
}
// get database connection
$database = new Database();
$db = $database->getConnection();
// prepare product object
$trivia = new Trivia($db);
// set ID property of record to read
$search = isset($_GET['id']) ? $_GET['id'] : die();
// read the details of product to be edited
$stmt = $trivia->getQuestion($search);
$num = $stmt->rowCount();
if($num > 0){
// create array
$questions_arr = array();
$questions_arr["records"] = array();
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)){
extract($row);
$questions_item = array(
"id" => $id,
"question" => $question,
"answer" => $answer,
"hint" => $hint,
);
array_push($questions_arr["records"], $questions_item);
}
// set response code - 200 OK
http_response_code(200);
// make it json format
echo json_encode($questions_arr);
}
else{
// set response code - 404 Not found
http_response_code(404);
// tell the user product does not exist
echo json_encode(array("message" => "no items found"));
}
?>
Notice that we are including database.php (step 1) and trivia.php (step 2) which are detailed in previous posts. If there are questions regarding getQuestion or similar code specifics answers can be found in previous “Building Your PHP REST Service” posts.
How do we process those items retrieved by the getQuestion function? First, we count the number to make sure that we have one (only one since we are looking for a specific question ID). Next we process the id/question/answer/hint into an array and encode it to be returned.
In step 5 and beyond, we will get to read unique counties and regions along with processing the information for those areas. That information includes name-address-phone plus social, review, mapping and web site links.