In the article for step 2, we continued building building our Representational State Transfer (REST) service by looking at files located in the objects directory.
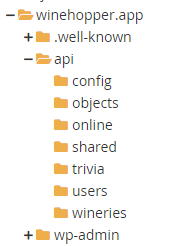
Next, we will look at the shared directory. Our final project, located at https://winehopper.app does not use this directory. Shared will contain a file such as utilities.php which contains a getPaging function which is shared among all WordPress PHP theme pages. That function is shown below.
<?php
class Utilities{
public function getPaging($page, $total_rows, $records_per_page, $page_url){
// paging array
$paging_arr=array();
// button for first page
$paging_arr["first"] = $page>1 ? "{$page_url}page=1" : "";
// count all products in the database to calculate total pages
$total_pages = ceil($total_rows / $records_per_page);
// range of links to show
$range = 2;
// display links to 'range of pages' around 'current page'
$initial_num = $page - $range;
$condition_limit_num = ($page + $range) + 1;
$paging_arr['pages']=array();
$page_count=0;
for($x=$initial_num; $x<$condition_limit_num; $x++){
// be sure '$x is greater than 0' AND 'less than or equal to the $total_pages'
if(($x > 0) && ($x <= $total_pages)){
$paging_arr['pages'][$page_count]["page"]=$x;
$paging_arr['pages'][$page_count]["url"]="{$page_url}page={$x}";
$paging_arr['pages'][$page_count]["current_page"] = $x==$page ? "yes" : "no";
$page_count++;
}
}
// button for last page
$paging_arr["last"] = $page<$total_pages ? "{$page_url}page={$total_pages}" : "";
// json format
return $paging_arr;
}
}
?>
In step 4, we will be able to explore the PHP code that retrieves a trivia question, hint and answer using a randomly selected ID.