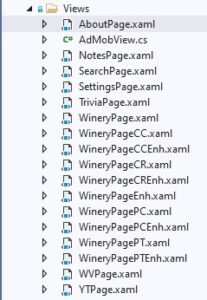
In this post, we will take a look at the AdMobView.cs and NotesPage used in our WetYourWhistle mobile app.
Why do we have a routine called AdMobView and why have you seen references to AdMob so far? Because everyone deserves to make a dollar or two from all of their programming efforts. AdMob is one of the ways to monetize your mobile app, creating income from ads placed in inconspicuous places.
The code shown below is included in our AdMobView.cs class. What is it doing? I am glad that you were wondering that. This public class inherits main functionality from, or is built upon, the standard View class. As illustrated, it is only creating two properties – AdUnitIdProperty and AdUnitId which allow posting of ad revenue to our AdMob account.
public class AdMobView : View
{
public static readonly BindableProperty AdUnitIdProperty = BindableProperty.Create(
nameof(AdUnitId),
typeof(string),
typeof(AdMobView),
string.Empty);
public string AdUnitId
{
get => (string)GetValue(AdUnitIdProperty);
set => SetValue(AdUnitIdProperty, value);
}
}
In NotesPage.xaml, we have omitted a few of the initial declarations previously shown in AboutPage.xaml. We have a toolbar item calls a procedure in NotesPage.cs, documented below. As in AboutPage, we are using two grid rows with the bottom defined with a height of 50 to display our ad and the top row consuming the rest of vertical screen height.
Next we have a stacklayout with a label and picker which enables you to rate the specific winery and create your own notes. Then we see the code which creates our AdMob ad block.
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage ...
xmlns:d="http://xamarin.com/schemas/2014/forms/design"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
...
mc:Ignorable="d"
x:Class="NYWineryHopper.Views.NotesPage"
Title="Notes">
<ContentPage.ToolbarItems >
<ToolbarItem Icon="QuestionMark_72.png" Clicked="LoadAbout" />
</ContentPage.ToolbarItems>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="*" />
<RowDefinition Height="50" />
</Grid.RowDefinitions>
<StackLayout Grid.Row="0">
<Label x:Name="lblNameLabel" FontSize="Medium" />
<Picker x:Name="pckRating" Title="How do you rate this winery?" FontSize="Large"> <!--SelectedIndexChanged="PckRating_SelectedIndexChanged" >-->
<Picker.ItemsSource>
<x:Array Type="{x:Type x:String}">
<x:String>1 Star, Not at all impressed</x:String>
<x:String>2 Stars, Room for improvement</x:String>
<x:String>3 Stars, Average</x:String>
<x:String>4 Stars, Almost perfect</x:String>
<x:String>5 Stars, Awesome</x:String>
</x:Array>
</Picker.ItemsSource>
</Picker>
<Editor x:Name="txtNotes" HeightRequest="200" />
<Button Text="Save" Clicked="SaveNotes"/>
</StackLayout>
<controls:MTAdView x:Name="mtAd" Grid.Row="1" />
</Grid>
</ContentPage>
What is happening here? As we had mentioned about AdMobView, the main functionality of this class is based on ContentPage. After declaring a few variables used in our procedure, we receive the Id being fed in and assign it to the wineId variable. Then we call the LoadNotes procedure.
In LoadNotes, we see that it is assigning variables by executing routines from App.Database and App.WineryManager. These were discussed in the Data Directory post. After assigning variable names to our screen items, those are populated from the saved data (if found).
In this C# code we have the procedure which will redirect to the AboutPage when the toolbar item is clicked. We also have the routine which will save the entered/edited data for the current winery.
[XamlCompilation(XamlCompilationOptions.Compile)]
public partial class NotesPage : ContentPage
{
int wineId;
int recordId;
string nameLabel;
public NotesPage(int Id)
{
InitializeComponent();
wineId = Id;
LoadNotes();
}
private async void LoadAbout(object sender, EventArgs e)
{
await Navigation.PushAsync(new AboutPage());
}
private async void SaveNotes(object sender, EventArgs e)
{
noteItem item = new noteItem();
var rate = this.FindByName<Picker>("pckRating");
var note = this.FindByName<Editor>("txtNotes");
if (recordId > 0)
item.Id = recordId;
item.recId = wineId;
item.Rating = rate.SelectedIndex + 1;
item.Note = note.Text;
var res = await App.Database.SaveItemAsync(item);
await Navigation.PopAsync();
}
private async void LoadNotes()
{
var item = await App.Database.GetItemAsync(wineId);
nameLabel = await App.WineryManager.GetName(wineId);
var rate = this.FindByName<Picker>("pckRating");
var lbl = this.FindByName<Label>("lblNameLabel");
var note = this.FindByName<Editor>("txtNotes");
lbl.Text = "Notes for " + nameLabel;
if (item != null)
{
rate.SelectedIndex = item.Rating - 1;
note.Text = item.Note;
recordId = item.Id;
}
}
}